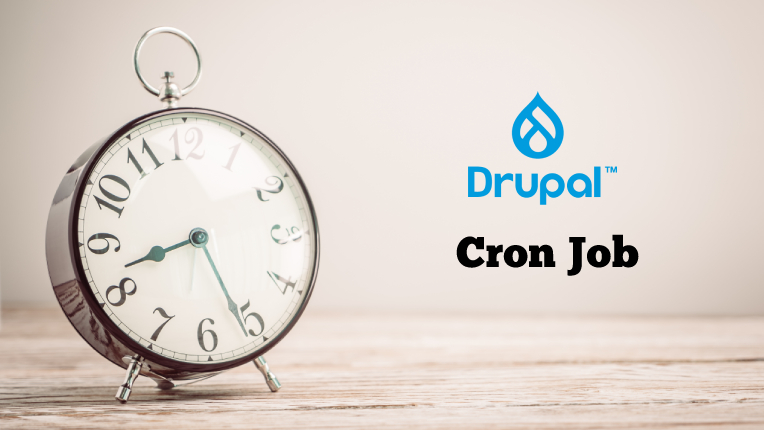
To add a once a day Cron run functionality in a custom module in Drupal 9, you can use Drupal's Cron API to schedule a task to run once a day. Here's an example code snippet that shows how to set up a once a day task:
<?php
use Drupal\Core\State\StateInterface;
use Drupal\Core\Datetime\DrupalDateTime;
use Drupal\Core\Logger\LoggerChannelFactoryInterface;
/**
* Implements hook_cron().
*/
function mymodule_cron() {
// Get the last run time of the task.
$state = \Drupal::service('state');
$last_run = $state->get('mymodule_daily_task_last_run');
// Check if the task has not run yet today.
$now = new DrupalDateTime();
$today = $now->format('Y-m-d');
if ($last_run != $today) {
// Update the last run time in state.
$state->set('mymodule_daily_task_last_run', $today);
// Schedule the task to run once a day.
\Drupal::service('cron')->addTask('mymodule_daily_task');
// Log that the task has been scheduled.
$logger = \Drupal::service('logger.factory')->get('mymodule');
$logger->notice('The daily task has been scheduled to run.');
}
}
/**
* Implements hook_cron_queue_info().
*/
function mymodule_cron_queue_info() {
$queues['mymodule_daily_task'] = [
'worker callback' => 'mymodule_daily_task_worker',
'time' => 86400, // 24 hours in seconds.
];
return $queues;
}
/**
* Worker callback for the daily task.
*/
function mymodule_daily_task_worker($data) {
// Perform the once a day task here.
// ...
// Log that the task has run.
$logger = \Drupal::service('logger.factory')->get('mymodule');
$logger->notice('The daily task has run.');
}
?>
In this code, we are using Drupal's State API to store the last run time of the task. We are also using Drupal's Logger API to log that the task has been scheduled and has run successfully.
The code checks if the task has not run yet today. If the last run time is not today's date, then we schedule the task to run once a day using the addTask() method of the CronInterface. We also update the last run time in state.
We define a queue for our once a day task using hook_cron_queue_info(). In the queue definition, we specify the worker callback function that will perform the task and the time interval (in seconds) for the task to be run again.
Finally, we define the worker callback function mymodule_daily_task_worker() that performs our once a day task.